Java 实现Gzip 压缩字符串
2020-01-10
阅读 {{counts.readCount}}
评论 {{counts.commentCount}}
前言
之前用JavaScript
实现过gzip压缩字符串的功能
链接: Javascript 实现Gzip 压缩字符串
这次是再用Java
实现一次Gzip对于字符串的压缩和解压缩
另外简单说明一下Gzip
1. Gzip
是一种压缩程序的格式,和zip
rar
7z
差不多,本文利用的是Gzip
把字符串压缩成二进制byte[]
2. 压缩后的内容会再用base64
编码变回base64字符串
方便传输和存储数据库
3. 压缩后的内容不一定比压缩前的要短,取决于重复的字符多不多,原文长度够不够长
4. 下文代码只用原生Java 8的内部依赖,只有一个外部依赖是StringUtils
也可以用Java8的语言来写,我只是懒得再写一遍
代码
import org.apache.commons.lang.StringUtils;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.util.Base64;
import java.util.zip.GZIPInputStream;
import java.util.zip.GZIPOutputStream;
/**
* @author zmh
* @date 2020-01-07
* <p>
* Gzip压缩字符串工具类
*/
public class GzipUtils {
public static final String GZIP_ENCODE_UTF_8 = "UTF-8";
/**
* base64 编码
*
* @param bytes 传入bytes
* @return 编码成string类型的base64返回
*/
public static String base64encode(byte[] bytes) {
return new String(Base64.getEncoder().encode(bytes));
}
/**
* base64 解码
*
* @param string 传入string类型的base64编码
* @return 解码成byte类型返回
*/
public static byte[] base64decode(String string) {
return Base64.getDecoder().decode(string);
}
/**
* 压缩字符串
*
* @param string 需要压缩的字符串
* @return 压缩后内容 并转base64 返回
*/
public static String gzip(String string) {
String result = "";
if (StringUtils.isBlank(string)) {
return result;
}
ByteArrayOutputStream out = new ByteArrayOutputStream();
GZIPOutputStream gzip;
try {
gzip = new GZIPOutputStream(out);
gzip.write(string.getBytes(GZIP_ENCODE_UTF_8));
gzip.close();
out.close();
result = base64encode(out.toByteArray());
} catch (Exception e) {
e.printStackTrace();
}
return result;
}
/**
* 解压缩字符串
*
* @param string base64格式的压缩后字符串
* @return 解码并解压缩后返回
*/
public static String unGzip(String string) {
String result = "";
if (StringUtils.isBlank(string)) {
return result;
}
ByteArrayOutputStream out = new ByteArrayOutputStream();
ByteArrayInputStream in;
GZIPInputStream ungzip;
byte[] bytes = base64decode(string);
try {
in = new ByteArrayInputStream(bytes);
ungzip = new GZIPInputStream(in);
byte[] buffer = new byte[1024];
int len = 0;
while ((len = ungzip.read(buffer)) != -1) {
out.write(buffer, 0, len);
}
ungzip.close();
out.close();
in.close();
result = out.toString(GZIP_ENCODE_UTF_8);
} catch (Exception e) {
e.printStackTrace();
}
return result;
}
public static void main(String[] args) {
long temp = System.currentTimeMillis();
String test = "Gzip压压压压缩缩缩缩缩测试测试测试测试测试试试试试试试试试试试aaaabbbbbccccccaaaabbbbbccccccaaaabbbbbccccccdddddd111111111111111111111111111111111111111";
String gzip = gzip(test);
String unGzip = unGzip(gzip);
System.out.println("原文:" + test);
System.out.println("Gzip压缩:" + gzip);
System.out.println("Gzip解压:" + unGzip);
System.out.println("整体消耗时间: " + (System.currentTimeMillis() - temp) + " ms");
}
}
执行结果
原文:Gzip压压压压缩缩缩缩缩测试测试测试测试测试试试试试试试试试试试aaaabbbbbccccccaaaabbbbbccccccaaaabbbbbccccccdddddd111111111111111111111111111111111111111
Gzip压缩:H4sIAAAAAAAAAHOvyix42tcNR8/3rERGz7Z2v1g/FReJHyUCQRIIJIMBfm4KGBgSBwDGzrxFtQAAAA==
Gzip解压:Gzip压压压压缩缩缩缩缩测试测试测试测试测试试试试试试试试试试试aaaabbbbbccccccaaaabbbbbccccccaaaabbbbbccccccdddddd111111111111111111111111111111111111111
整体消耗时间: 39 ms
END
补充:经测试,发现Gzip
的压缩率其实挺高,但仅限于压缩成Byte[]
,如果再把Byte[]
转成base64,会增加大小,所以这里可以根据需求,来考虑是否可以直接用二进制存储和传输
评论区空空如也,赶紧添加一条评论吧
评论 {{counts.commentCount}}

{{comment.name}}
{{comment.os}}
{{comment.browser}}
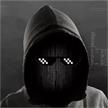
{{comment.reply.name}}
{{comment.reply.os}}
{{comment.reply.browser}}
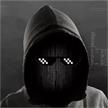