前后端高效率实现Base64编码解码
2018-08-18
阅读 {{counts.readCount}}
评论 {{counts.commentCount}}
前端javascript没什么可说的啦 直接贴代码吧
- // 引入base64.js
- <script type="text/javascript" src="js/base64.js"></script>
- // base64.js 文件内容
- function Base64() {
- // private property
- _keyStr = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/=";
- // public method for encoding
- this.encode = function (input) {
- var output = "";
- var chr1, chr2, chr3, enc1, enc2, enc3, enc4;
- var i = 0;
- input = _utf8_encode(input);
- while (i < input.length) {
- chr1 = input.charCodeAt(i++);
- chr2 = input.charCodeAt(i++);
- chr3 = input.charCodeAt(i++);
- enc1 = chr1 >> 2;
- enc2 = ((chr1 & 3) << 4) | (chr2 >> 4);
- enc3 = ((chr2 & 15) << 2) | (chr3 >> 6);
- enc4 = chr3 & 63;
- if (isNaN(chr2)) {
- enc3 = enc4 = 64;
- } else if (isNaN(chr3)) {
- enc4 = 64;
- }
- output = output +
- _keyStr.charAt(enc1) + _keyStr.charAt(enc2) +
- _keyStr.charAt(enc3) + _keyStr.charAt(enc4);
- }
- return output;
- }
- // public method for decoding
- this.decode = function (input) {
- var output = "";
- var chr1, chr2, chr3;
- var enc1, enc2, enc3, enc4;
- var i = 0;
- input = input.replace(/[^A-Za-z0-9\+\/\=]/g, "");
- while (i < input.length) {
- enc1 = _keyStr.indexOf(input.charAt(i++));
- enc2 = _keyStr.indexOf(input.charAt(i++));
- enc3 = _keyStr.indexOf(input.charAt(i++));
- enc4 = _keyStr.indexOf(input.charAt(i++));
- chr1 = (enc1 << 2) | (enc2 >> 4);
- chr2 = ((enc2 & 15) << 4) | (enc3 >> 2);
- chr3 = ((enc3 & 3) << 6) | enc4;
- output = output + String.fromCharCode(chr1);
- if (enc3 != 64) {
- output = output + String.fromCharCode(chr2);
- }
- if (enc4 != 64) {
- output = output + String.fromCharCode(chr3);
- }
- }
- output = _utf8_decode(output);
- return output;
- }
- // private method for UTF-8 encoding
- _utf8_encode = function (string) {
- string = string.replace(/\r\n/g,"\n");
- var utftext = "";
- for (var n = 0; n < string.length; n++) {
- var c = string.charCodeAt(n);
- if (c < 128) {
- utftext += String.fromCharCode(c);
- } else if((c > 127) && (c < 2048)) {
- utftext += String.fromCharCode((c >> 6) | 192);
- utftext += String.fromCharCode((c & 63) | 128);
- } else {
- utftext += String.fromCharCode((c >> 12) | 224);
- utftext += String.fromCharCode(((c >> 6) & 63) | 128);
- utftext += String.fromCharCode((c & 63) | 128);
- }
- }
- return utftext;
- }
- // private method for UTF-8 decoding
- _utf8_decode = function (utftext) {
- var string = "";
- var i = 0;
- var c = c1 = c2 = 0;
- while ( i < utftext.length ) {
- c = utftext.charCodeAt(i);
- if (c < 128) {
- string += String.fromCharCode(c);
- i++;
- } else if((c > 191) && (c < 224)) {
- c2 = utftext.charCodeAt(i+1);
- string += String.fromCharCode(((c & 31) << 6) | (c2 & 63));
- i += 2;
- } else {
- c2 = utftext.charCodeAt(i+1);
- c3 = utftext.charCodeAt(i+2);
- string += String.fromCharCode(((c & 15) << 12) | ((c2 & 63) << 6) | (c3 & 63));
- i += 3;
- }
- }
- return string;
- }
- }
- // 用法:先实例化
- var base = new Base64();
- // 加密
- var xxx = base.encode(xxx);
- // 解密
- var xxx = base.decode(xxx);
后端就厉害了,我找了3种方法实现,最后才发现,最好用的,效率最高的,其实就是java8自带的。。。
只贴java8自带的代码了
- // 引入依赖必须选java.util.Base64
- import java.util.Base64;
- public class BASE64Utils{
- // 已经直接写成了最简单直接的方法,丢在工具类 ,无脑调用就行
- public static String base64Encode(String text) throws IOException{
- return Base64.getEncoder().encodeToString(text.getBytes("UTF-8"));
- }
- public static String base64Decode(String text) throws IOException{
- return new String(Base64.getDecoder().decode(text), "UTF-8");
- }
- }
- // 例如工具类叫BASE64Utils
- // 加密
- String xxx = BASE64Utils.base64Encode("xxx");
- // 解密
- String xxx = BASE64Utils.base64Decode("xxx");
大功告成啦 若有问题可以留言哈~
评论区空空如也,赶紧添加一条评论吧
评论 {{counts.commentCount}}

{{comment.name}}
{{comment.os}}
{{comment.browser}}
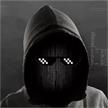
{{comment.reply.name}}
{{comment.reply.os}}
{{comment.reply.browser}}
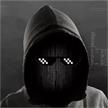